Save code that works
It takes hours to find a code snippet or tutorial that actually works. And double that when you search for it again. We help you save & access snippets in less than 5 secs with our web app, Chrome & IDE extensions. Just right-click and save!
arrow_forward Get startedSave code snippets
that work for the next time you need them
Save to the cloud
Access your snippets across devices and never lose them
Organize snippets
Group similar snippets together in collections or by hashtags
Save with a Chrome Extension
Don't lose that snippet you found on Stack Overflow after trying a dozen different snippets
Save in Visual Studio Code
Instantly access & save your snippets while you code using keyboard shortcuts
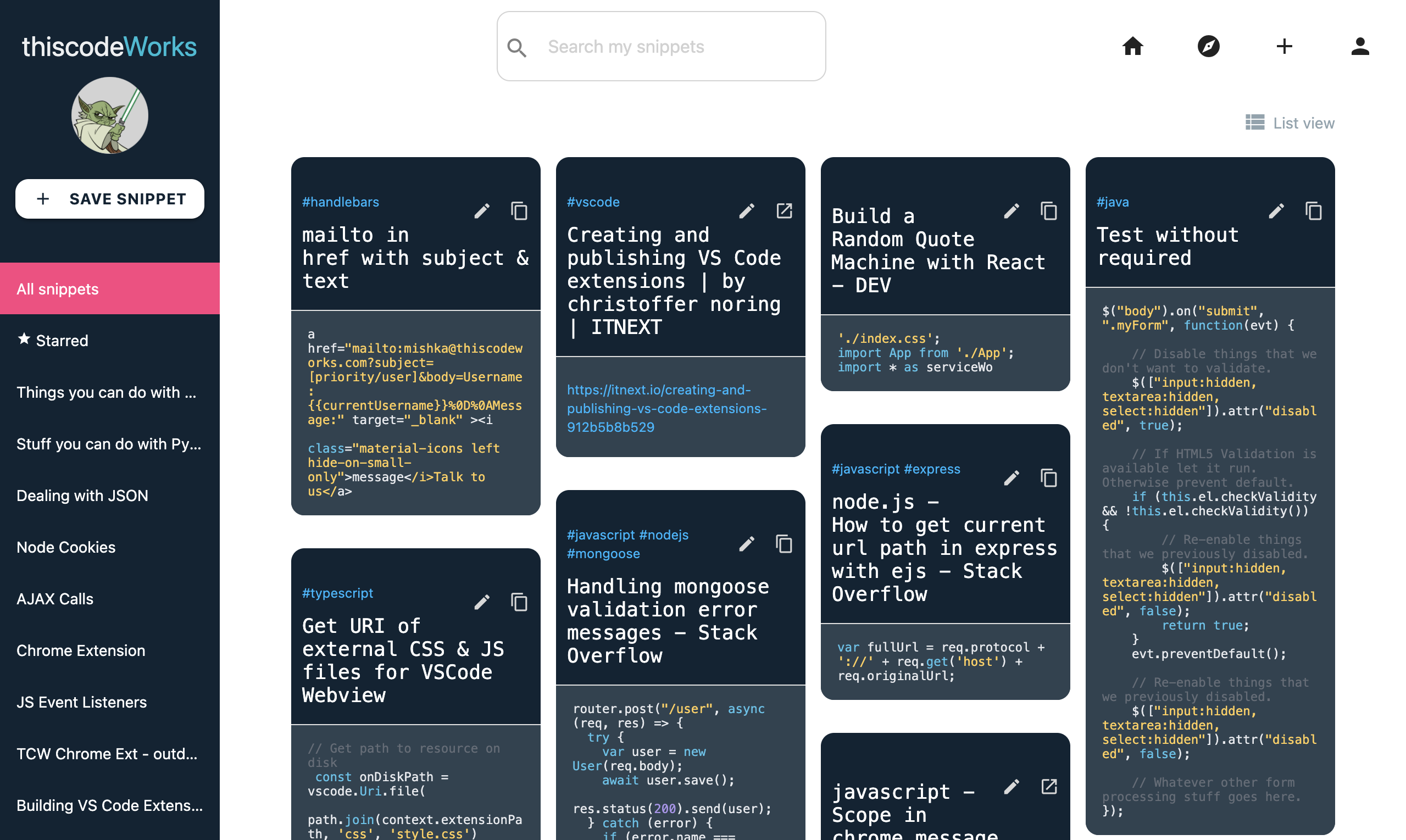
Share code snippets
Every snippet you save has multiple ways to share
Explore the Pinterest of Code
Discover new snippets & collections saved by the community